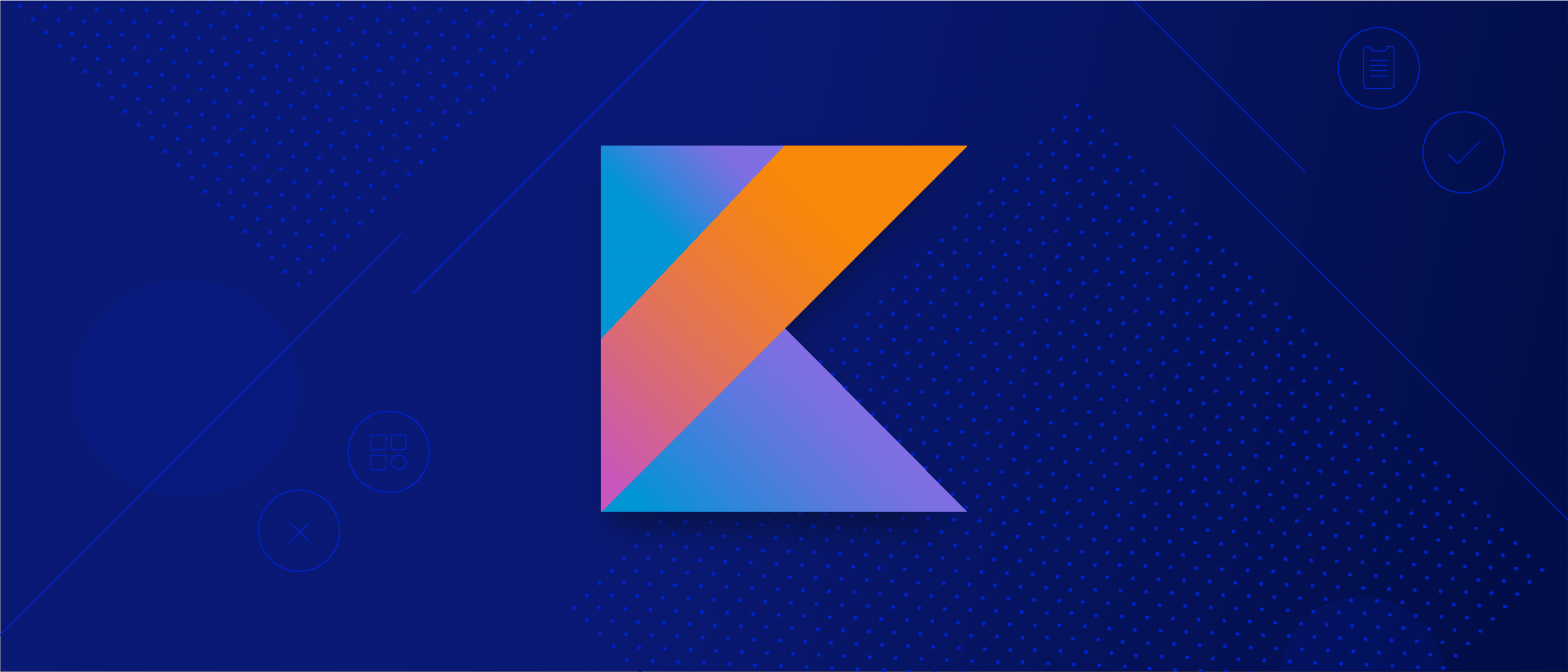
Why should I as a Java developer consider moving to Kotlin?
Some Java issues addressed in Kotlin
- Null references are controlled by the type system.
- No raw types
- Arrays in Kotlin are invariant
- Kotlin has proper function types, as opposed to Java’s SAM-conversions
- Use-site variance without wildcards
- Kotlin does not have checked exceptions
What Java has that Kotlin does not
- Checked exceptions
- Primitive types that are not classes
- Static members
- Non-private fields
- Wildcard-types
- Ternary-operator a ? b : c
What Kotlin has that Java does not
- Lambda expressions + Inline functions = performant custom control structures
- Extension functions
- Null-safety
- Smart casts
- String templates
- Properties
- Primary constructors
- First-class delegation
- Type inference for variable and property types
- Singletons
- Declaration-site variance & Type projections
- Range expressions
- Operator overloading
- Companion objects
- Data classes
- Separate interfaces for read-only and mutable collections
- Coroutines
Ok, cool, sounds like Kotlin has quite some benefits comparing to Java. Where would I start?
Operators and basic constructs of the language
There are some differences from the coding perspective when it comes to usage of Kotlin.
Unit – analogue of void in Java
infix – make available for inline execution aka x <operator> y;
operator infix – can also be replaced with a real operator, aka +;
tailrec MUST be used for recursive calls where applicable;
Classes, Interfaces and OOP
Classes and methods by default are final, the user will need to specify that class or method is allowed to be overridden or extended explicitly with ‘open‘ keyword.
Everything is public by default. No package-private scope BUT Kotlin has internal visibility scope. This will mean that the object is accessible within a module: IntelliJ, Eclipse, maven, Gradle, whatever module. Also has protected and private. But does not have Java-specific package access.
Sealed classes provide a way of restricting class hierarchy to only known registered classes. In general looks like enum on steroids.
Constructors are defined right after the class name. Can contain val-s and var-s. Under the hood, these are converted to properties with the private access and appropriate accessors for ‘get’ and/or ‘set’. Constructor parameters can also not belong to class:
class Person(_name: String) { val name = _name }
We can also define secondary constructor using constructor keyword. But in general, it’s not a best-practice. It’s better to avoid secondary constructors in favour of default values in the primary constructor.
Data classes also support copy operation with named parameters to override values
Companion object allows you to define static methods. But in fact, those are static only in Kotlin world. To make them also status in Java-world, you will need to add annotation @JvmStatic, like the following:
companion object { @JvmStatic fun main(args: Array<String>) {} }
No Comments